Developing a school management system using Python and Django is a complex but rewarding project. It involves various components like student management, course scheduling, attendance tracking, and more. Here’s a detailed plan to help you get started:
1. Project Setup:
- Set up a virtual environment and install Django.
- Create a new Django project and app for the school management system.
- Configure project settings, including database setup.
2. Design Database Models:
- Define database models for essential entities like students, teachers, courses, classes, attendance, grades, and more.
- Establish relationships between models using ForeignKey, OneToOneField, or ManyToManyField.
3. Create Templates:
- Design user interface templates for different sections of the school management system using HTML, CSS, and JavaScript.
- Utilize a frontend framework or library like Bootstrap to streamline the UI design.
4. User Authentication and Authorization:
- Implement user registration and login features for students, teachers, and administrators.
- Set up different user roles and permissions to control access to different sections of the system.
5. Student Management:
- Allow administrators to add, edit, and delete student records.
- Provide a dashboard for students to view their details, classes, grades, and attendance.
6. Teacher Management:
- Enable administrators to manage teacher records, including personal details and assigned courses.
- Allow teachers to view and manage their assigned courses and classes.
7. Course Management:
- Create a system to define and manage courses offered by the school.
- Allow administrators to assign teachers to specific courses.
8. Class and Schedule Management:
- Design a schedule system to assign courses to classes and manage class timings.
- Allow teachers and students to view their class schedules.
9. Attendance Tracking:
- Develop a mechanism to mark and track attendance for classes.
- Allow teachers and administrators to generate attendance reports.
10. Grade Management:
- Implement a grading system to record and manage student grades for assignments, exams, and quizzes.
- Allow teachers to input grades and students to view their grades.
11. Communication Features:
- Incorporate messaging functionality between students, teachers, and administrators.
- Implement notifications for important updates, events, and deadlines.
12. Reporting and Analytics:
- Create reports for attendance, grades, and other relevant data.
- Implement data visualization tools to present data in a comprehensible manner.
13. Testing:
- Perform thorough testing at each stage of development to identify and fix bugs.
- Implement automated tests using Django’s testing framework.
14. Deployment:
- Deploy the school management system to a hosting platform such as Heroku or a VPS provider.
- Set up the production environment, including database configuration and static files handling.
15. User Training and Documentation:
- Provide user guides or documentation for administrators, teachers, and students to understand how to use the system effectively.
16. Maintenance and Updates:
- Monitor the system after deployment and address any issues or bugs that arise.
- Consider adding new features and making improvements based on user feedback.
We have to keep in mind and remember that building a complete school management system is a substantial project that might require collaboration with others. Prioritize modular and maintainable code, and consider using version control systems like Git to manage your project’s codebase. Additionally, Django’s official documentation and online tutorials can provide valuable guidance throughout the development process.
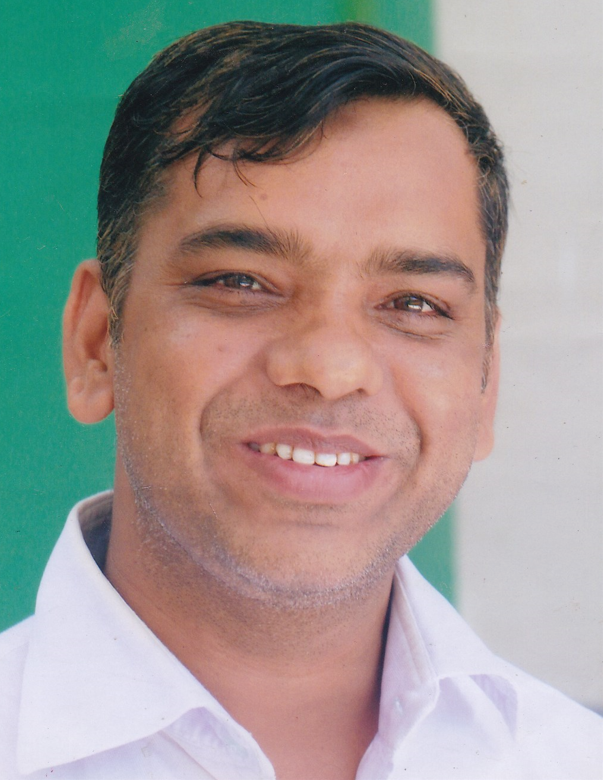
Sayed is a Backend Python Programmer at sayed.xyz with 1+ years of experience in tech. He is passionate about helping people become better coders and climbing the ranks in their careers, as well as his own, through continued learning of leadership techniques and software best practices.