Compiled Languages:
A compiled language like C or C++ requires the source code to be transformed into machine code before it can be executed by the computer’s CPU. This transformation is done using a compiler, which takes the entire source code as input and produces an executable binary file. The binary file contains the machine code that the computer can directly understand and execute.
Advantages of Compiled Languages:
Generally, compiled code can execute faster because it is directly translated into machine code optimized for the target hardware.
Compilation checks for any errors before the code is executed, leading to potentially more robust software.
Disadvantages of Compiled Languages:
The compilation process can be time-consuming, especially for large programs.
Compiled programs are often platform-specific, requiring different executables for different operating systems or architectures.
Interpreted Languages:
An interpreted language like Python is executed line by line by an interpreter, which reads the source code and executes it directly without the need for a separate compilation step. The interpreter translates the source code into machine code or bytecode (an intermediate representation) on the fly, executing each line as it encounters it.
Advantages of Interpreted Languages:
Interpreted languages are generally more portable, as a single source code can be executed on different platforms without modification.
Development and testing cycles can be faster since there’s no need to wait for compilation.
Disadvantages of Interpreted Languages:
Interpreted code can be slower than compiled code due to the runtime translation process.
Errors are often detected only when the interpreter encounters the problematic line, potentially leading to runtime issues.
Python’s Approach:
Python is often categorized as an “interpreted” language, but it’s more accurate to describe it as a “bytecode compiled” language. Here’s why:
When you run a Python script, the source code is first translated into bytecode by the Python interpreter. This bytecode is a lower-level representation of the code that can be executed more efficiently than the original source code. The bytecode is then executed by the Python interpreter, making it appear as if the code is being interpreted.
We can discuss it more precisely that Python is often referred to as an “interpreted” language because it uses an interpreter to execute code, but it actually employs a hybrid approach. When you run a Python script, the source code is first compiled into bytecode, which is then executed by the Python interpreter. This bytecode allows for faster execution than pure interpretation and provides a compromise between the advantages of compiled and interpreted languages.
Key points about Python’s approach:
Bytecode Compilation:
Python source code is compiled into bytecode before execution. This compilation step makes the execution process more efficient than interpreting the source code line by line.
Interpreter:
The bytecode is executed by the Python interpreter. The interpreter reads and executes the bytecode instructions, making decisions and performing actions accordingly.
Dynamic Typing:
Python is dynamically typed, which means the type of a variable is determined at runtime. This is a characteristic often associated with interpreted languages.
Interactive Mode:
Python’s interactive mode allows you to enter code directly and see the results immediately, which is more typical of interpreted languages.
In summary, while compiled languages transform source code into machine code before execution, interpreted languages execute source code directly using an interpreter. Python falls somewhere in between with its bytecode compilation approach, combining elements of both paradigms.
In another way, we can say that Python combines aspects of both compiled and interpreted languages. It compiles the source code into bytecode before execution, but it still relies on an interpreter to execute the bytecode. This hybrid approach provides a balance between execution speed and developer convenience.
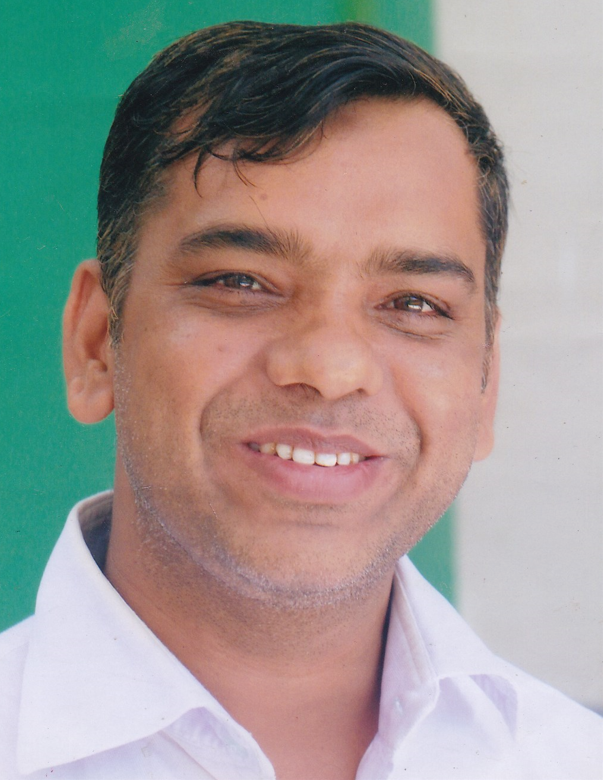
Sayed is a Backend Python Programmer at sayed.xyz with 1+ years of experience in tech. He is passionate about helping people become better coders and climbing the ranks in their careers, as well as his own, through continued learning of leadership techniques and software best practices.