Natural Language Processing (NLP) is a field of artificial intelligence that focuses on enabling computers to understand, interpret, and generate human language. Python offers powerful libraries and tools for NLP tasks.
Here’s a step-by-step guide to getting started with NLP using Python:
Basics of Python:
Make sure you’re familiar with Python’s syntax, data structures, and programming concepts. This foundation will be crucial for working with NLP libraries.
Text Preprocessing:
Before analyzing text, you need to preprocess it:
- Tokenization: Split text into words or sentences.
- Stopword Removal: Eliminate common words like “the,” “and,” “is,” etc.
- Stemming and Lemmatization: Reduce words to their base or root form.
NLTK (Natural Language Toolkit):
- NLTK is a comprehensive library for NLP tasks. Install it using pip.
- Explore NLTK’s functionalities for text processing, tokenization, stemming, and more.
- Use NLTK’s corpora and resources for text analysis.
Text Analysis:
Perform basic text analysis tasks like word frequency, n-grams, and part-of-speech tagging.
Identify named entities (people, organizations, locations) using NLTK’s named entity recognition.
Text Classification:
Learn about supervised learning algorithms for text classification.
Use libraries like Scikit-learn to implement classification tasks such as sentiment analysis, spam detection, etc.
Sentiment Analysis:
Analyze sentiment in text using pre-trained sentiment analysis models or train your own.
Topic Modeling:
Understand topic modeling algorithms like Latent Dirichlet Allocation (LDA).
Use libraries like Gensim to perform topic modeling on text data.
Word Embeddings:
Learn about word embeddings like Word2Vec and GloVe.
Use libraries like Gensim or spaCy to work with pre-trained word embeddings.
spaCy:
spaCy is another popular NLP library that’s known for its speed and efficiency.
Explore spaCy’s capabilities for tokenization, named entity recognition, and part-of-speech tagging.
Text Generation:
Understand techniques for text generation, including Markov chains and recurrent neural networks (RNNs).
Experiment with generating text using libraries like TensorFlow or PyTorch.
Advanced Topics:
Depending on your interests, explore more advanced NLP topics:
i) Neural Language Models: Explore models like Transformer and BERT for advanced language understanding.
ii) Machine Translation: Implement machine translation using models like Seq2Seq.
iii) Named Entity Recognition (NER): Learn how to extract structured information from text.
Real-World Projects:
Apply your NLP skills to real-world projects, such as building chatbots, analyzing social media data, or extracting insights from large text corpora.
Community and Learning:
Participate in NLP communities, read research papers, and take online courses to stay updated with the latest NLP advancements.
Remember that NLP is a vast field with a wide range of applications. The key to mastering NLP using Python is hands-on practice, experimentation, and continuous learning.
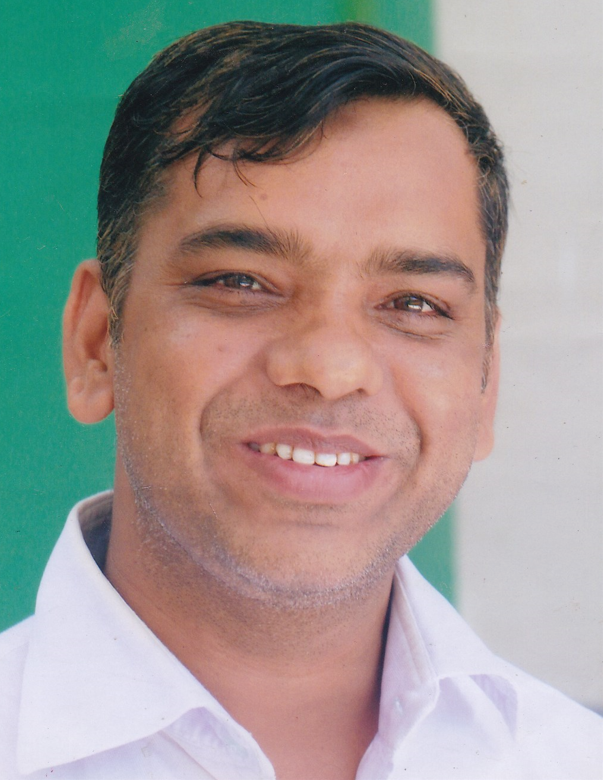
Sayed is a Backend Python Programmer at sayed.xyz with 1+ years of experience in tech. He is passionate about helping people become better coders and climbing the ranks in their careers, as well as his own, through continued learning of leadership techniques and software best practices.