A full Python course typically covers a wide range of topics, starting from the basics and progressing to more advanced concepts.
Here’s an outline of what you might expect in a comprehensive Python course:
Module 1: Introduction to Python
1.1. What is Python?
- Introduction to Python’s history and popularity.
- Installation and setup of Python and a code editor/IDE.
1.2. Python Basics
- Variables and data types (integers, floats, strings, booleans).
- Basic operations (arithmetic, string manipulation, comparison).
- Printing and commenting code.
1.3. Control Flow
- Conditional statements (if, elif, else).
- Loops (for and while).
- Logical operators (and, or, not).
1.4. Functions
- Defining and calling functions.
- Parameters and return values.
- Scope and namespaces.
Module 2: Data Structures in Python
2.1. Lists
- Creating and manipulating lists.
- List methods and slicing.
2.2. Tuples
- Creating and working with tuples.
- Immutable nature of tuples.
2.3. Dictionaries
- Creating and accessing dictionaries.
- Dictionary methods and operations.
2.4. Sets
- Creating and using sets.
- Set operations (union, intersection, etc.).
Module 3: Object-Oriented Programming (OOP) in Python
3.1. Classes and Objects
- Defining classes and creating objects.
- Attributes and methods.
3.2. Inheritance and Polymorphism
- Inheritance and subclassing.
- Method overriding and polymorphism.
3.3. Encapsulation and Abstraction
- Access modifiers (public, private, protected).
- Abstract classes and interfaces.
Module 4: Error Handling and Exception Handling
4.1. Exception Handling
- Understanding exceptions.
- Handling exceptions with try-except blocks.
4.2. Custom Exceptions
- Creating custom exception classes.
- Raising exceptions.
Module 5: File Handling
5.1. Reading and Writing Files
- Opening and closing files.
- Reading from and writing to files.
5.2. Working with File Paths
- Manipulating file paths using the
os
module.
Module 6: Python Modules and Libraries
6.1. Introduction to Modules
- Importing and using modules.
- Creating your own modules.
6.2. Popular Python Libraries
- Exploring commonly used libraries like
numpy
,pandas
, andmatplotlib
(data science) orFlask
andDjango
(web development).
Module 7: Introduction to Functional Programming
7.1. Lambda Functions
- Creating and using lambda functions.
7.2. Map, Filter, and Reduce
- Functional programming concepts in Python.
Module 8: Working with Data (Optional)
8.1. Data Serialization (JSON, XML, etc.)
- Reading and writing structured data formats.
8.2. Database Connectivity (SQL or NoSQL)
- Basic database operations in Python.
Module 9: Advanced Topics (Optional)
9.1. Multithreading and Multiprocessing
- Concurrency in Python.
9.2. Decorators and Generators
- Advanced Python features.
Module 10: Final Project
10.1. Building a Real-World Project – Applying knowledge gained throughout the course to build a Python application.
10.2. Testing and Debugging – Best practices for testing and debugging Python code.
This is a comprehensive outline of what a full Python course may cover. Depending on the course’s duration and depth, certain topics may be covered in more detail, and additional advanced topics may be introduced. Additionally, hands-on exercises, coding projects, and quizzes are typically included to reinforce learning.
Additional advanced topics:
In addition to the core topics covered in a comprehensive Python course, there are several advanced topics and specialized areas of Python programming that you may explore to become a more proficient Python developer. Here are some additional advanced topics you can consider:
1. Web Development with Python:
- Web Frameworks: Learn popular Python web frameworks like Django and Flask to build web applications.
- RESTful APIs: Understand how to create and consume RESTful APIs for building web services.
- Front-End Integration: Explore frontend libraries and frameworks like React, Angular, or Vue.js for building modern web applications.
2. Data Science and Machine Learning:
- NumPy and SciPy: Dive deeper into numerical and scientific computing using these libraries.
- Pandas: Master data manipulation and analysis with Pandas.
- Scikit-Learn: Learn about machine learning algorithms and data modeling.
- Deep Learning: Study deep learning frameworks like TensorFlow and PyTorch.
- Data Visualization: Explore data visualization libraries like Matplotlib and Seaborn.
3. Data Analysis and Data Engineering:
- Data Pipelines: Learn about data processing pipelines using tools like Apache Spark and Apache Kafka.
- Big Data: Explore big data technologies like Hadoop and HBase for large-scale data processing.
- Database Systems: Deepen your knowledge of SQL and NoSQL databases (e.g., PostgreSQL, MongoDB).
4. DevOps and Deployment:
- Docker and Containers: Understand containerization and Docker for packaging and deploying applications.
- Continuous Integration/Continuous Deployment (CI/CD): Implement CI/CD pipelines for automated testing and deployment.
- Cloud Services: Explore cloud platforms like AWS, Azure, or Google Cloud for deploying and scaling applications.
5. Cybersecurity:
- Ethical Hacking: Study cybersecurity practices and ethical hacking techniques using Python.
- Cryptography: Learn about encryption and decryption using Python libraries.
6. Automation and Scripting:
- Scripting for System Administration: Automate system tasks and administrative processes.
- GUI Automation: Use tools like Selenium for web automation and PyAutoGUI for GUI automation.
7. Game Development:
- Pygame: Explore game development using the Pygame library.
- 3D Graphics: Learn about 3D graphics programming using libraries like PyOpenGL.
8. IoT (Internet of Things):
- MicroPython: Program microcontrollers and IoT devices using MicroPython.
9. Natural Language Processing (NLP):
- NLTK (Natural Language Toolkit) and spaCy: Analyze and process natural language text data.
- Text Classification: Build text classification models for sentiment analysis and more.
10. Advanced Python Topics:
- Metaclasses: Study advanced object-oriented programming concepts.
- Concurrency and Parallelism: Learn about advanced concurrency mechanisms like asyncio.
- Python Performance Optimization: Optimize Python code for better performance using profiling and Cython.
These advanced topics will depend on your specific interests and career goals. It’s a good idea to explore the areas that align with your career aspirations and project requirements. Remember that practical experience through projects and real-world applications is essential for mastering these advanced topics.
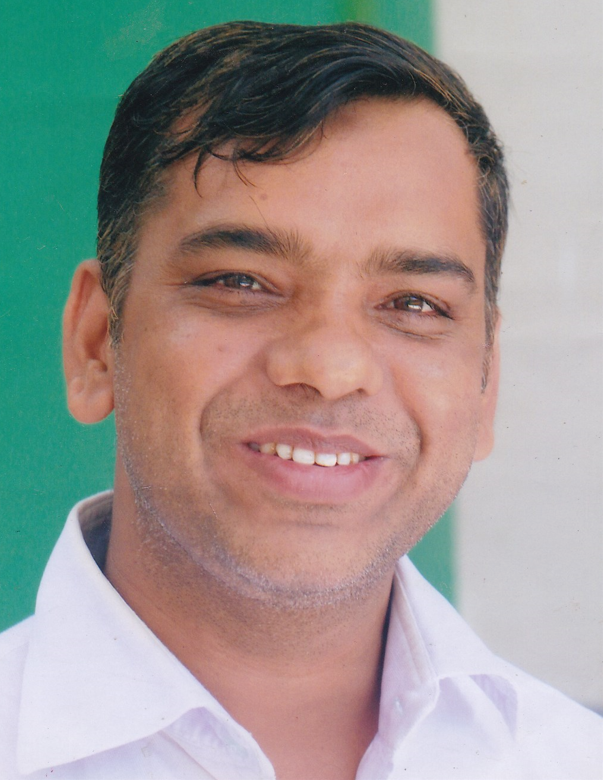
Sayed is a Backend Python Programmer at sayed.xyz with 1+ years of experience in tech. He is passionate about helping people become better coders and climbing the ranks in their careers, as well as his own, through continued learning of leadership techniques and software best practices.