Python is an excellent choice for scripting and automation due to its simple syntax, wide range of libraries, and cross-platform compatibility. Whether you’re automating repetitive tasks, managing files, or interacting with APIs, Python can help streamline your workflow. Here’s a guide to scripting and automation using Python:
Basics of Python:
Make sure you have a solid understanding of Python’s fundamentals, including variables, data types, loops, conditional statements, functions, and error handling.
Identify Tasks for Automation:
Identify repetitive tasks or processes that can be automated. This could include tasks like file manipulation, data extraction, report generation, and more.
Working with Files and Directories:
Python’s built-in libraries make it easy to work with files and directories:
Use the os module to perform file and directory operations.
The shutil module can help with more advanced file operations like copying, moving, and deleting.
Web Scraping and APIs:
Use libraries like requests or urllib to interact with websites and APIs.
Parse HTML using libraries like BeautifulSoup or work with JSON using the built-in json module.
Regular Expressions:
If you need to search for and manipulate patterns in strings, learn how to use regular expressions with Python’s re-module.
Automating Tasks:
Use the subprocess module to run external processes and programs.
Automate tasks involving other applications, such as opening files with the default viewer.
Scheduled Tasks:
Use the time and schedule libraries to schedule scripts to run at specific times.
Email Automation:
Use the built-in smtplib and email modules to send automated emails.
Fetch emails from an inbox using the imaplib module.
Data Processing and Analysis:
Automate data processing tasks using libraries like Pandas and NumPy.
Create scripts that preprocess and analyze data without manual intervention.
Interacting with Databases:
Use libraries like sqlite3 or higher-level ORM libraries like SQLAlchemy to automate database interactions.
Testing and Debugging:
Write unit tests to ensure your scripts are working as intended.
Use debugging tools and techniques to identify and fix errors.
Version Control:
Use version control systems like Git to keep track of changes to your scripts.
Documentation:
Comment your code thoroughly and create clear documentation, especially if others will be using or maintaining your scripts.
Security and Error Handling:
Be mindful of security concerns when automating tasks, especially if they involve sensitive data or actions.
Implement error handling to gracefully handle unexpected issues.
Sharing and Distribution:
Package your scripts into standalone executables using tools like pyinstaller.
Share your scripts with others, keeping in mind licensing and usage terms.
Remember that scripting and automation can save you significant time and effort, but it’s important to thoroughly test your scripts and ensure they are robust before deploying them in production environments.
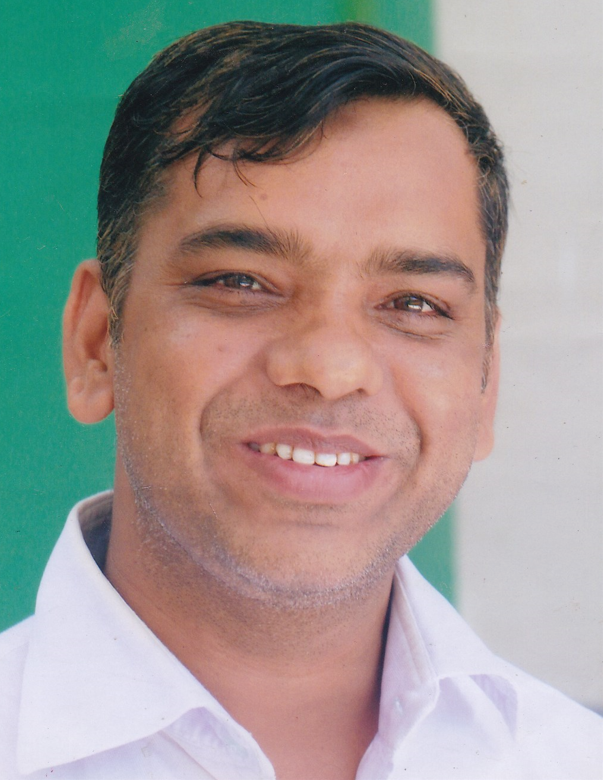
Sayed is a Backend Python Programmer at sayed.xyz with 1+ years of experience in tech. He is passionate about helping people become better coders and climbing the ranks in their careers, as well as his own, through continued learning of leadership techniques and software best practices.